Introduction to Licenses
License support in MIP
Introduction
The MIP license system supports two different schemes for how a partner plug-in implements a license system.
Generally, there are these possible types of license schemes:
- A site-level license system
- A system that counts partner plug-in known objects
It is also possible to work in trial mode; in this case, the plug-in defines the trial period and how many connections or machines that can run during this trial period.
A plug-in developer needs to consider how to handle the following when defining a license scheme for a plug-in:
- New connections
- New users
- Trial mode
- How long to accept users in trial mode
XProtect License and Plug-in Licenses
An XProtect license is identified by a Software License Code (SLC). Plugin licenses are added to the SLC as a sub-product and cannot be used on any other system. Plug-in licenses are identified by the Partner company name, a plug-in ID and a license ID.
Activation Process
In the Management Client, you can activate licenses online or offline. In addition, all MIP plug-ins provide their license requests and receive their license responses from the license server. There are no fields or forms to be filled in during this process.
Implementation
This section describes how the activation process has been implemented and the classes that are involved.
- Users running the Management Client select the online license activation.
- The application retrieves a list of license requests from all MIP plug-ins.
- The application embeds the MIP plug-in license information in its normal license information and signs and encrypts the license request.
- The application sends the request to the Milestone license server.
- The Milestone license server validates the request based on what is registered on the given SLC. All MIP plug-in sub-products are checked.
- The Milestone server builds A license response is built from the base SLC information, the normal camera count, information, and trial expiry dates.
- All checked MIP plug-ins and information are added to the base license.
- The license is signed and encrypted and sent back to the application that sent the request.
- The application will decrypt and validate the signature.
- If OK, the license is stored locally and MIP plug-ins receive the new license.
License Check Schemes
The MIP Environment enables two ways of implementing license checks; it is up to the specific plug-in developer to decide how the license check should work for that plug-in:
- Site license – when a license with a counter of 1 is activated, the plug-in is licensed .
- Reserved licenses – the license counter checks the number of items that can be created and reserves an individual license for each item.
Trial Modes
Independent of the license method, a trial mode should also be considered. A trial mode can be applied for the entire license (relevant for site method), or for individual reserved licenses when adding items above and beyond what the current activated license covers.
Suggested trial modes:
- Site license: have a trial license running for 30 days.
- Reserved licenses: Allows two items running in 14–30 days. The two items would continually be two more than the number of already licensed items. This allows users to add new items from time to time and then activate them afterwards.
User Interaction
The user administrating the system can get an overview of the license status by clicking on the License Information icon. An overview page with all loaded plug-ins and their license status is displayed.
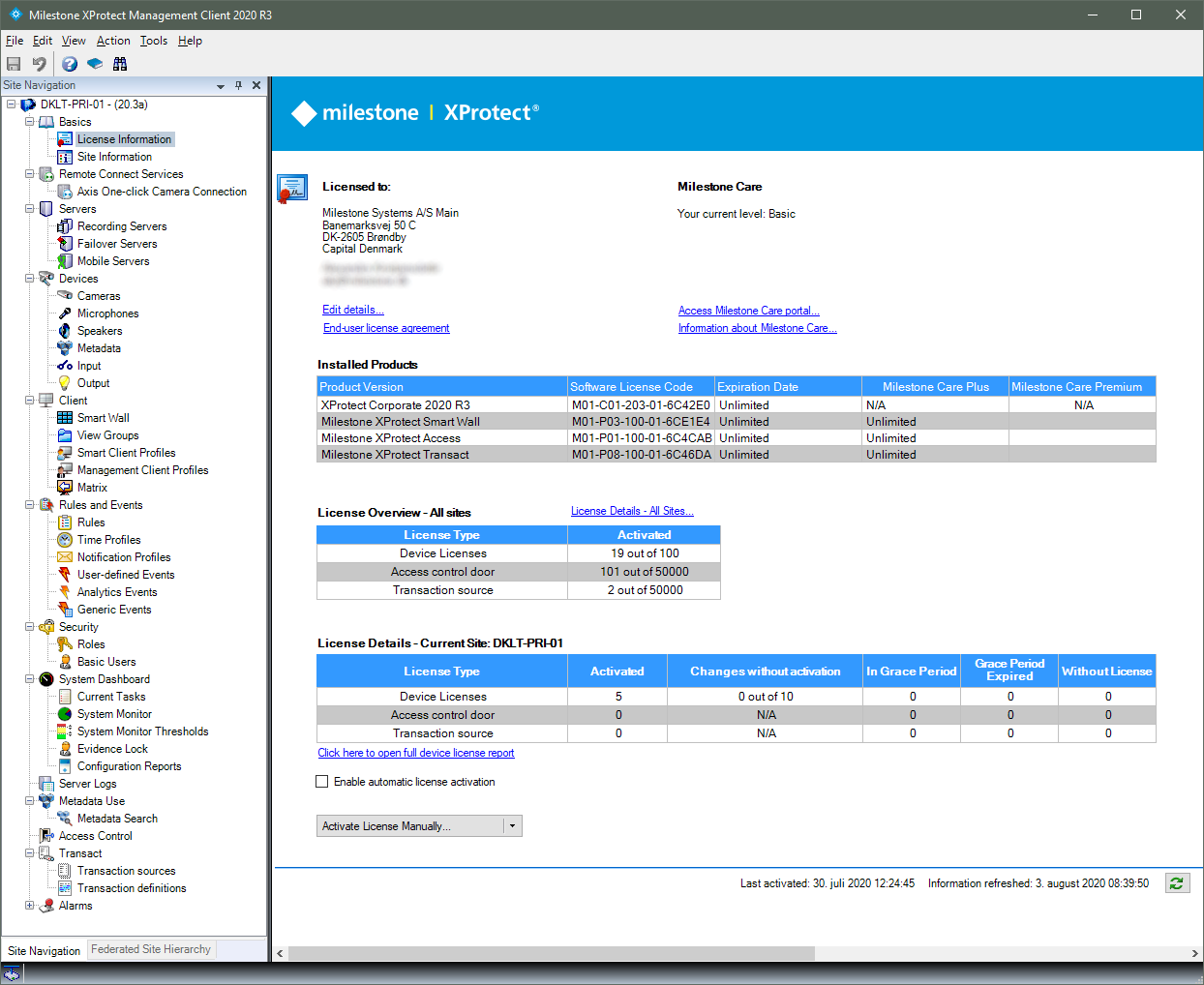
When using reserved licenses, the license status should be displayed on the plug-in’s overview page, help page, or on the configuration page for each item.
MIP Environment Supplied Classes & Methods
SystemLicense
The SystemLicense
class contains information located in the XProtect system
license. It can be accessed from the
EnvironmentManager.Instance.SystemLicense
.
public class SystemLicense
{
public virtual string SLC { get; internal set; }
public virtual DateTime Issued { get; internal set; }
public virtual DateTime Expire { get; internal set; }
public virtual int GracePeriod { get; internal set; }
}
LicenseManager
The LicenseManager
class contains a set of methods and properties to assist a
MIP plug-in in controlling and validating the selected license scheme used in the
plug-in:
- The
GetLicense()
method is used to get the last activated license for the plugin. - The
ReservedLicenseManager
class is used for reserving licenses for specific items, for example, server connections to a plug-in owned server.
public class LicenseManager
{
public Collection<LicenseInformation> GetLicense(Guid pluginid);
public LicenseInformation GetLicense(Guid pluginid, string licenseType);
public ReservedLicenseManager ReservedLicenseManager;
}
ReservedLicenseManager
The ReservedLicenseManager
class provides save and get methods for saving the
activated or trial licenses. When a license has been saved with this method, it can
be accessed from Smart Client or standalone applications.
The ReservedLicenseManager
class works with the LicenseInformation
class, which also has a number of methods to store the individual reserved
licenseItems. For each license that is reserved for a given item, one entry should
be registered within the LicenseInformation
class.
MIP Plug-in Classes & Methods to Be Implemented
PluginDefinition
A property in the PluginDefinition
defines whether the plugin has a license scheme.
public override Collection<License.LicenseInformation> PluginLicenseRequest
The method does not have to be implemented if no license scheme is being used for this
plugin. If there is a license scheme, the most updated
LicenseInformation
must be returned . The property is accessed when the
Managment Client/Application activates a license (online or offline).
LicenseInformation
The LicenseInformation
contains the license itself. It is generated by the
plug-in and returned to the MIP Environment during an activation request, and the
same type of class is returned when it has been activated on the license server. The
key fields that are checked on the license server are the PluginId
, the
LicenseType
, and the Counter
fields. Upon returning the
activated LicenseInformation
, the counter value is modified to the
number of licenses bought. The trial mode, the expiry date, and the
CustomData
fields can be modified.
If the license server cannot find any bought licenses for this plug-in (relating to the given SLC), the counter is set to zero.
ItemManager
The ItemManager
class can implement license support by adding code to:
Init()
: Get the saved licenses or create an initial trial license.Init()
: Register a message receiver to be notified when new licenses are activated.MessageHandler
: Receive new licenses, handle changes, and store licenses for remote retrieval.
When reserving licenses to specific items, the following code must be added:
- Add/CreateItem: Validate if new items are allowed; register the item.
- Delete: Unregister the deleted item and possibly transfer licenses from deleted items to other items.
UserControls
The Management client and the user should be able to see if a plug-in is running on a trial license, if it is disabled because of a missing license, or if a trial period has expired. When reserving license for items, the displayed content should be close to where the configuration is done, or in an overview/help page.
MIP License spanning multiple sites
Please review the LicenseInformation
class documentation for MIP licenses while reading this section.
License in MFA
The license request must include the number of requested licenses – or optionally the requested license items. If the license or any of the license items are in trial mode, this should also be indicated in the request.
Note: If the license is a non-activated trial license, the number of available trial licenses can be indicated by specifying a license count different from the number of requested license items, and setting CounterMethod
to CounterMethodEnum.CountByItemIdentifications
.
Example 1: Request by count
var licenseRequest = new LicenseInformation()
{
PluginId = _pluginId,
LicenseType = _licenseType,
Name = _licenseName,
Counter = 3,
CounterMethod = LicenseInformation.CounterMethodEnum.CountByCounter,
CustomData = "",
};
Example 2: Request by license items
var licenseRequest = new LicenseInformation()
{
PluginId = _pluginId,
LicenseType = _licenseType,
Name = _licenseName,
Counter = 3,
ItemIdentifications = new Collection<LicenseItem>()
{
new LicenseItem() { Id = "itemId1" },
new LicenseItem() { Id = "itemId2" },
new LicenseItem() { Id = "itemId3" },
},
CounterMethod = LicenseInformation.CounterMethodEnum.CountByItemIdentifications,
CustomData = "",
};
The license response will include the total license count as well as the number of activated licenses on the site.
Furthermore, if the request included license items, the response will include those items that have been activated on the site. License items will always be activated in the order they appear in the collection, and non-activated items will be removed from the collection. Finally, the ItemTrial flag will be unset on all activated license items, indicating that these have been activated and are no longer in trial.
var licenseResponse = EnvironmentManager.Instance.LicenseManager.GetLicense(_pluginId, _licenseType) as LicenseResponse;
if (licenseResponse != null)
{
// licenseResponse.Counter - Total number of licenses
// licenseResponse.Activated - Number of activated licenses on this site
// licenseResponse.ItemIdentifications - Activated license items on this site
}
else
{
// License not activated
}