Plug-in development
Prerequisites
We expect you use Visual Studio 2017, 2019, or 2022. All plug-in samples have a solution file and are targeting .NET Framework 4.7.
A licensed version of an XProtect Video Management System should be installed on the machine that you use for debugging. If you are developing a plug-in for the XProtect Smart Client, you should make sure that the XProtect Smart Client is installed. You can use the standard released products and there are no requirements for installing debug versions or special components.
Milestone MIP plug-ins should be compiled as Any CPU or x64 and can be run in 64-bit architecture.
Process
The following gets you started with the C# development for a Milestone MIP plug-in:
Install Milestone MIP SDK Templates Visual Studio extension.
Install XProtect VMS. The following products can be used:
- XProtect Express+
- XProtect Professional+
- XProtect Expert
- XProtect Corporate
You can get 30-day trial licenses from https://www.milestonesys.com/products/software/try-xprotect/.
Do not use versions that are no longer supported. Please check the Milestone Product Lifecycle page at https://www.milestonesys.com/support/tools-and-references/product-lifecycle
Create a new project in Visual Studio, and select the right MIP SDK plug-in project template.
In the following example, the project name ABC
is used. When you enter ABC
, the following solution is created:
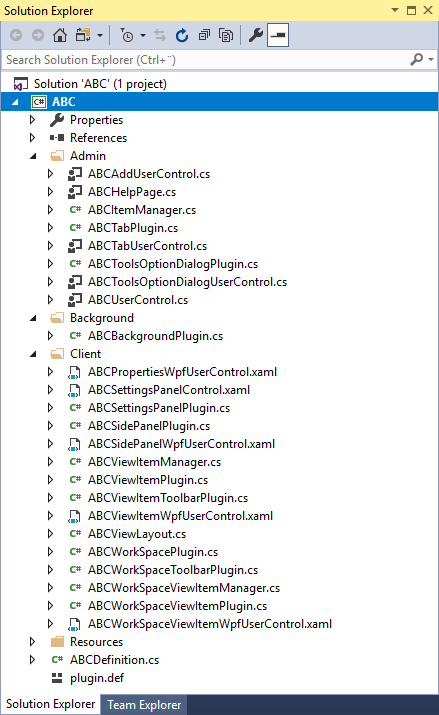
All class names are prefixed with the project name, and new GUIDs are generated. You now have a set of files as well as a project and a solution file that can compile.
To run the plug-in, copy the file ABC.DLL
and the plugin.def
file to the shared plug-in folder on the machine (%PROGRAMFILES%\Milestone\MIPPlugins\"your-plug-in-name"\
)
All MIP-enabled applications will load plug-ins from this place, unless the plug-in is found in the application-specific MIPPlugins
folder first – see the following section.
Plug-in Location
The folders used for loading the plug-ins are named MIPPlugins
. The folders are placed as follows:
Location | |
---|---|
Executing folder of the starting application | .\MIPPlugins |
A common place that all Milestone and OEM applications load from | %PROGRAMFILES%\VideoOS\MIPPlugins |
A common place that all Milestone applications load from | %PROGRAMFILES%\Milestone\MIPPlugins |
For XProtect Smart Client – Player, if the player is not included with the exported video data, the plug-ins are loaded only from the executing folder of the XProtect Smart Client.
If the export contains the player, the plug-ins with PluginDefinition.IncludeInExport
property set to true are included in the export and they are loaded from the Client Files\Client\MIPPlugins
folder of the export.
VideoOS
is used as an alternative company name to support OEM versions of any XProtect products. If you want your plugin to be supported by these OEM versions, place your plug-in under the %PROGRAMFILES%\VideoOS\MIPPlugins
folder. For backwards compatibility, we will continue to load plug-ins from %PROGRAMFILES%\Milestone\MIPPlugins
as well.
For the XProtect Smart Client, the folder structure looks like this:
For the XProtect Management Client and the XProtect Event Server, you can create a similar folder named MIPPlugins
with sub-folders for each plug-in.
The plugin.def
file defines which DLL to load. This is done to avoid loading irrelevant DLLs.
<plugin>
<file name="ServerSideCarrousel.dll" />
<load env="Client, Administration" />
</plugin>
The load
element defines in which environments the plug-in should be loaded. If the load
element is not present, the plug-in will be loaded in all applications.
The value of the attribute env
contains the EnvironmentType
enumerations as defined in the VideoOS.Platform.EnvironmentManager
. Currently, these value are supported:
Administration
Client
(same asSmartClient
;Client
is supported from Smart Client 2020 R3)SmartClient
Standalone
Service
The order of loading is:
- First, the plug-ins that are located in the application-specific folders are loaded.
- Then the plug-ins in the shared folder are loaded.
If duplicates exist, the application-specific plug-in becomes the active one.
Verify that the plug-in is loaded
It can be verified that a Smart Client plug-in is loaded by using the Help About dialog. You find this in the top right-hand corner of the Smart Client: the icon with a question mark. Click on the question mark icon, and in the drop-down menu select About. The dialog looks like this:
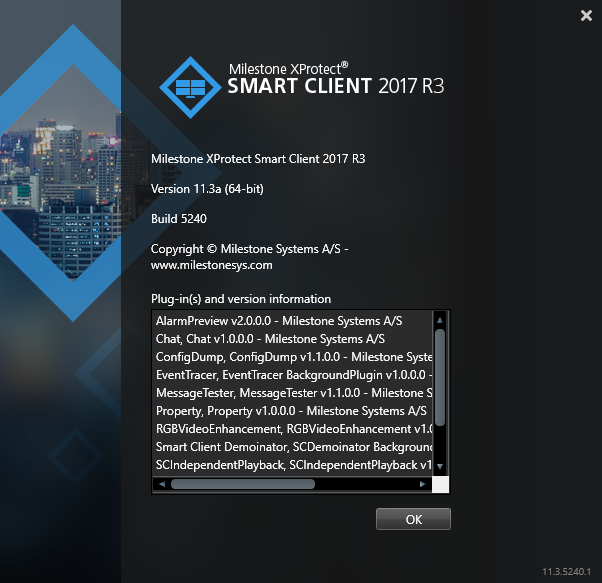
To verify the plug-in is loaded in the Management Client, open the Help > About dialog:
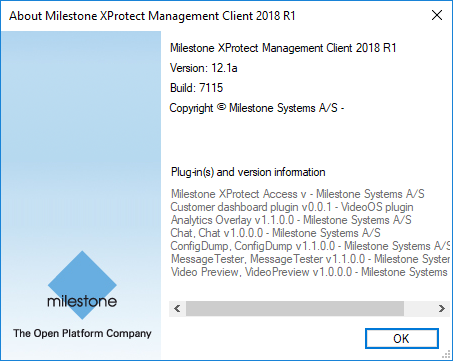
To find if an Event Server plug-in is loaded, you must find the last time the Event Server was started, or alternatively, make an Event Server restart, and then after the restart, examine today’s log.
In the MIP logs, look for the PluginLoader
information:
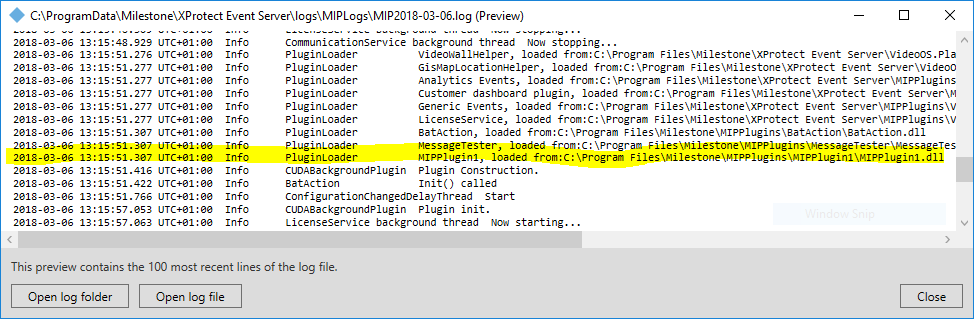
Debug logging
By using the VideoOS.Platform.EnvironmentManager.Log
method, you can output debugging text to the MIP log. An example of this is:
EnvironmentManager.Instance.Log(true, "SCLicenseSample", ex.Message);
If you need general information about when your plug-in is loaded and similar, use this method (but remember to remove it before widely distributing the plug-in, because it will affect all plug-ins running in the Smart Client):
EnvironmentManager.Instance.TraceFunctionCalls = true;
When logging is used, it is important to note that the Smart Client logging per default is turned off.
Enable logging in the Smart Client
- Start the Smart Client.
- Go to Settings.
- Go to the Advanced tab.
- Switch Logging (for technical support) to Enabled.
Note, the way to do this has changed over time, older versions of the Smart Client have other instructions. For a complete coverage, and for troubleshooting tips, please see this knowledge base article.
Special note for Event Server
To enable debug logging from the Event Server, change the VideoOS.Event.Server.Exe.Config
file, located in C:\Program Files\Milestone\XProtect Event Server
:
<!--
Set MIP log level to either "debug", "info", or "error".
Set the "archive" attribute to change the MIP log path. Default path is "MIPLogs"
under the event server log directory.
-->
<MIPLoggerSettings level="info" />
Change the level from info to debug to enable debug relevant messages in the log. Then restart the Event Server.
When developing plug-ins for the Access Control module, modify this part in the above file:
<!-- The access control trace level. Traces all calls and events between the Event Server and the plugin. The traces is found in the MIP log files. Valid values are: 'Disabled', 'Simple' and 'Detailed'. -->
<add key = "AccessControlTraceLevel" value = "Disabled" />
Change the value to Simple
or Detailed
to get trace information written into the MIP log file.
Log File Locations
MIP environment | Path |
---|---|
XProtect Management Client | %ProgramData%\Milestone\XProtect Management Client\Logs\MIPtrace.Log |
XProtect Smart Client | %ProgramData%\Milestone\MIPlog.txt |
XProtect Event Server | %ProgramData%\Milestone\XProtect Event Server\logs\MIPLogs\MIPyyyy-MM-dd.log %ProgramData%\Milestone\XProtect Event Server\logs\Cyyyy-MM-dd.log |
XProtect Event Server comes with a log viewer, which makes the job easier. Find the Tray Manager (tray icon) for the Event Server, right-click and select Show MIP logs:
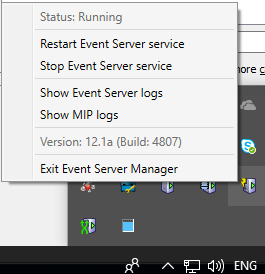
Event Server viewer displaying a MIP Log:
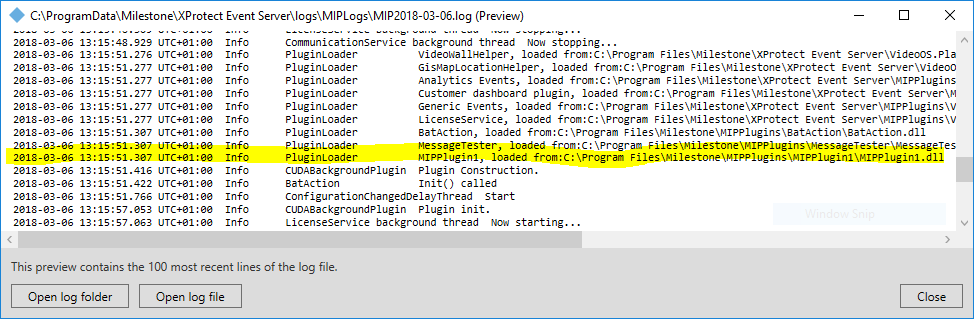
Start Debugging from Visual Studio
Place the compiled DLLs in the folder that is loaded by the application. You may want to make a copy statement in the Post-build event command line on the Build-Events tab within Visual Studio (see note 1).
Create a
plugin.def
file and place it in the same folder.Change Visual Studio project properties for
Debug
Change the Start external program so that it matches the application that should start (for the XProtect Smart Client, it would be
Client.exe
in the XProtect Smart Client install folder) (see note 2)For starting services, add the argument
-x
to the command line. (Make sure the service is stopped before you start the service exe file).
Make a build event (note 1)
When you want to debug the plug-in running in the Smart Client/Management Client/Event Server environment, it is important to ensure the newest build is loaded. To ensure this, it is recommended to make a Post-build event command line that copies the DLL files.
xcopy /y "$(TargetPath)" "C:\Program Files\Milestone\MIPPlugins\MIPPLugin1"
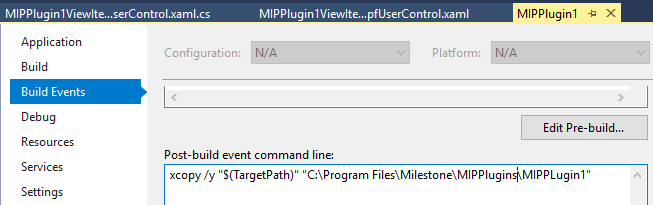
Note that, for this to work, you must make sure the Smart Client/Management Client/Event Server is stopped so that the file is not locked, and for Visual Studio to copy to Program Files
, it must be run as administrator.
The plugin.def
could also be copied automatically like this, but this file usually does not change, so you can just copy it once, manually.
Start the debugging (note 2)
If your plug-in is running in the Smart Client, you can start debugging using the Attach to Process debug option in Visual Studio. Attach to the Client.exe
process. You can make a break point in your code, and when it is hit, inspect the variables, single-step etc.
The drawback with this method is that, if what you want to debug is happening during initialization or similar, then you might never see it. It might have passed because you attach the debugger at a later point in time.
A better method is changing the Start action in your project to be Start external program and point to the Client.exe
:
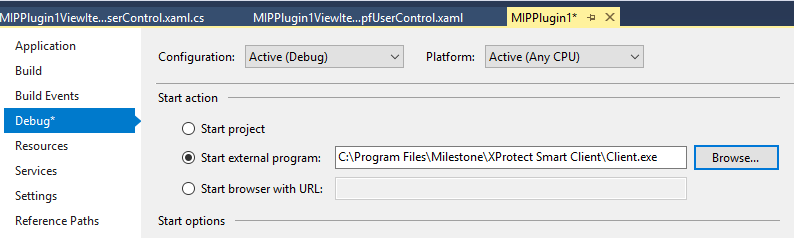
Similar for Management Client plug-ins:
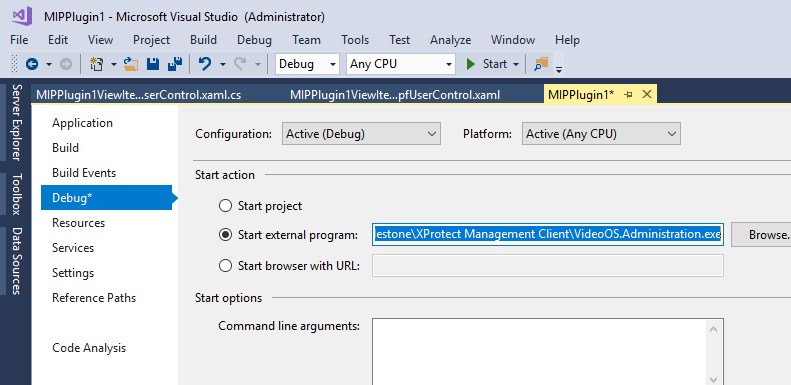
For an Event Server plug-in, you must stop the Event Server service, and start it as a Windows application from Visual Studio by supplying an -x
command line argument:
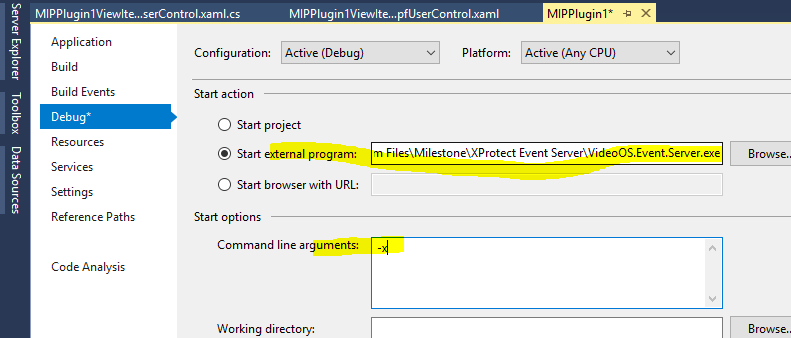
Deploy and Install
When you create a deployment project, the minimal set of files to include are the two files represented in the illustration below:
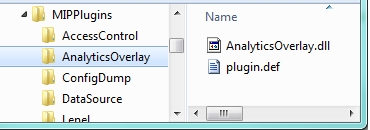
The DLL that you have developed and the .DEF
file that defines what to load. These two files, and any additional files that you may need, should be installed to the following folder:
%PROGRAMFILES%\VideoOS\MIPPlugins\"your-plug-in-name"\
( or %PROGRAMFILES%\Milestone\MIPPlugins\"your-plug-in-name"\ )
When located in this folder, the application loads the plug-in and executes relevant classes if the EnvironmentType matches the one that is defined in the plug-in definition. For plug-ins used by the XProtect Smart Client, the installer must be run on each computer where an XProtect Smart Client uses the plug-in.
Note: Re-start the Event Server service, if the plug-in needs to execute with the Event Server. For example, if the plug-in contains a specific background plug-in for the Event Server or if the plug-in provides access to items or devices to be used on the Smart Client Map and Alarm overview.
Version Strategy
The version of the MIP SDK and the version of the installed XProtect VMS at a customer site will often be out of sync, but it will work fine when:
- The plug-in only refers to one or more of the files that starts with "
VideoOS.Platform
" - The plug-in doesn't access functionality that was introduced in a later VMS version than the one used